When you start a new software project, you should think about how to write readable, clean code. PHP code should be easy to maintain and fix if needed and to achieve that, you should follow some basic standards and best practices. One of them is writing clean code that is easy to understand to others. How to write clean PHP code, then?
What are clean code principles?
Clean code principles are general guidelines that help developers write understandable, clean, and clear code that is easy to work on for other programmers. In most cases, PHP developers suggest the following to make your code clean:
- Don’t write PHP code as a single file from top to bottom. Use Classes or Functions.
- Use type declarations so that no one has to guess what a variable contains.
- Add simple underscores to parameter names so that others can tell which are private and protected, and which are public.
- Use value objects for immutability.
- Use logic inversion and early returning to avoid too many indentation levels and get rid of the else keyword.
- Avoid using else… or else. In most cases, the keyword is a code smell that tells that a developer is doing more than one thing in the method or section of code.
Simple tips to make your PHP code look better
Above, you can find some important principles set by experienced developers. However, there are also some best practices you should consider while writing PHP code.
- Your variables should have proper names so that everyone can easily see what they contain.
- Constants are better than hardcoded strings or numbers. Adding hardcoded strings or numbers can lead to strange and hard-to-debug bugs.
- Keep methods and functions short. Readability is much better when methods have 2-3 lines.
- Return early. It makes your PHP code cleaner and much easier to understand. On the other hand, nesting too deep makes your code less readable.
- Give your functions descriptive names so that their names clearly describe what they do.
- Don’t use too many comments. A bad comment can be confusing for a developer not aware of all the class purposes.
- Flags are not parameters. Each function or method should do only one thing well. Using a flag parameter means that a method does more than one thing based on the value of that flag.
- Tests are as important as production code. Therefore, don’t care less about good practices in the unit tests than in production code. Unit tests make your code maintainable and flexible, after all.
PHP language usage
PHP, one of the most popular programming languages, has been around for over 20 years. It’s considered to be a powerful and reliable solution, as well as the core technology for building comprehensive IT solutions. Some people say that PHP has been recently losing its popularity in favor of more recent languages. Is it true? Let’s explain how PHP is used now and why it remains popular. We’ll also compare it with other competitive development tools.
What is the PHP language and who uses it?
PHP is an open-source scripting language used for web development. The name of the language stands for Hypertext Preprocessor (initially, it stood for Personal Homepage) – it denotes a server-side language. It means that solutions written on it run on web servers and don’t depend on the web browser. The first version of the language was launched in 1995 (it was created a year before by Rasmus Lerdorf) and now it’s on version 8 released in November 2020. The initial version of PHP was a set of Perl scripts that was used to monitor traffic on Lerdorf’s website. The number of visitors was rapidly growing so Lerdoft decided to rewrite the scripts in C-language, adding more features.
Today, PHP is the most widely used open-source and general-purpose server-side scripting language. It is used by approximately 80% of websites worldwide. It runs on the Zend engine, which is the most popular implementation. The language is mostly used for making web servers. It can do anything related to server-side scripting (the backend of the website). It can receive data from forms, generate dynamic page content, create sessions, send and receive cookies, and much more.
The popular websites that use PHP:
- Facebook, Slack, and other tech giants.
- CMS solutions like WordPress or Drupal.
- E-commerce platforms like Magento.
- Web hosting platforms like BlueHost or Whogohost.
- Other websites like Wikipedia built in PHP, or MailChimp.
PHP usage today
Despite the fact that PHP’s popularity has been slowing down in the last few years, it’s still one of the most popular languages for backend development. It’s mainly because WordPress and Shopify which constitute around 45% of all websites today. According to statistics, roughly 244 million websites ran PHP at the end of 2021. PHP is usually used for website development but it’s extremely versatile. It is able to combine with other programming languages smoothly. It has a large number of available libraries and frameworks that extend PHP’s capabilities even further. The language can be used on all major operating systems including macOS, Linux, Microsoft Windows, many Unix variants, and RISC OS. On top of that, it has support for most of the web servers today. The three main areas where PHP scripts are used are server-side scripting (the most traditional target field for the language), command-line scripting, and writing desktop applications.
As already mentioned, the most famous examples of software written on PHP are WordPress and Facebook. WordPress is the most popular CMS on the net mostly used to create blogs, photo galleries, online stores, news portals, etc. On the other hand, Facebook is the most popular social network with a diverse range of functions.
The main advantages of PHP
Let’s dive deeper into the main benefits of using PHP for web development:
- It’s open-source and has a large community. Therefore, developers can learn faster and implement the latest solutions as early as possible – the vast community of PHP programmers is essential.
- PHP is perfectly scalable which is great if you are looking forward to the project’s growth. Your PHP-based website can be easily extended by adding more servers when needed.
- The language is cost-efficient. You don’t need any additional expensive software to work with. Also, you don’t need any extra licenses or royalty fees.
- It offers high speed. The latest PHP versions utilize memory well enough to optimize program execution speed. This way, response time is significantly reduced.
- PHP allows custom development. PHP’s wide use and pre-written code in composer libraries make developing functional applications much easier. When you pair the language with JavaScript, you can smoothly build highly functional and attractive websites customized to the client’s needs.
- PHP is cross-platform which makes it platform-independent. You don’t need a particular operating system to use it because the language runs on every platform.
- It has plentiful documentation. No language has so many tutorials, manuals, and other materials available. The documentation facilitates the development process and provides help and a source of inspiration when needed.
- Impressive selection of databases. PHP allows connections to almost any type of database. Of course, MySQL is the most common, but PHP is also compatible with mSQL, SQLite, PostgreSQL, etc. The language can be even used with Redis, MongoDB, ElasticSearch, and other non-relational databases.
- PHP is perfectly compatible with HTML and with cloud services.
- Finally, it’s flexible which makes it possible to combine with many other programming languages.
Are there any disadvantages of PHP?
Are there any cons, then? Of course, PHP, like everything, also has disadvantages. There are three main drawbacks:
- Sometimes, there are security problems, mainly because of the open-source nature of the language. It makes the language vulnerable, and developers with malicious intent may use vulnerabilities.
- Lack of specialized libraries. Of course, PHP has an extensive set of libraries, but it cannot compete with e.g., Python in developing web apps empowered by machine learning.
- The language doesn’t allow change or modification in the core behavior of online applications.
PHP vs. Python and Ruby
PHP is often compared with Ruby and Python. Python still trails far behind PHP in use for web development, however, it’s more flexible and easier than PHP. For now, it doesn’t yet offer the same level of database connectivity and support as PHP. Also, it has fewer frameworks to support rapid development. On the other hand, Ruby is famous for its elegant syntax and robust performance. However, it’s far more difficult to learn than PHP and it doesn’t offer the same extensive community support. The language you choose should then depend on the project you want to build and the features you want to prioritize.
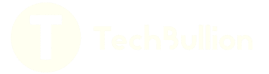