Before we start learning how to use arrays in PHP, let’s first learn what an array is in a nutshell. So let’s start.
What is an array in PHP?
Array is nothing but a special variable that has the capacity to hold multiple values. Each value has a corresponding key, which is used to access the value when we use an array.
How do you make an array in PHP or declare an array in PHP?
<?php
$colors=array(“red”,”green”,”yellow”,”black”);
echo “Colours are: $colors[0], $colors[1], $colors[2], and $colors[3]”;
?>
Output will be: Colours are: red, green, yellow and black
Types of Arrays?
So, what are the main types of arrays?
- Indexed Array
- Associative Array
- Multidimensional Array
What is an Indexed Array? An index array is a collection of numbers.
Learn React Hooks here is a more efficient way.
Index array example:
$colours[0] = “Red”;
$colours[1] = “Green”;
$colours[2] = “Blue”;
What is an Associative Array? Associative Arrays hold values with named keys.
Associative Array Example:
$age[‘Robin’] = “32”;
$age[‘Stroke’] = “27”;
$age[‘John’] = “33”;
What is a Multidimensional Array? A multidimensional array can contain multiple arrays or nested arrays.
Multidimensional Array Example: –
$name= array (
array(“Stroke”,22,”stroke@email.com”),
array(“Robin”,23,”robin@email.com”),
array(“Yusaf”,25,”yusaf@email.com”),
array(“Butler”,20,”butler@email.com”)
);
What are the benefits of using arrays in any language?
- You always end up coding less.
- When necessary, you can traverse all elements of an array using the key, because the index or key holds the value.
- You can accept multiple values from the form input.
- Sorting is damn easy with Array.
Array Sort Functions in PHP
- sort(): The sort function sorts arrays in ascending order.
- rsort(): The rsort function sorts arrays in descending order.
- asort(): The asort function is used to sort associative arrays in ascending order, according to the value
- ksort(): The ksort function is used to sort associative arrays in ascending order, according to the key
- arsort(): The arsort function is used to sort associative arrays in descending order, according to the value
- krsort(): The krsort function is used to sort associative arrays in descending order, according to the key
Does Array have predefined functions?
Yes, arrays provide lots of predefined functions. These functions are ready-made; no hard work is required; simply use them and pass parameters based on your needs to achieve the desired result. functions that are frequently used; we will discuss them in this article.
- array_combine() :- Returns the values from a single column in the input array.
- array() :- This function is used to create and return array.
- array_filter() : -Filters the values of an array using a callback function.
- array_key_exists() :-Checks if the given key exists in array or not.
- array_map() :- Sends each value of an array to a user-made function, which returns new values.
- array_merge() :- merge the given two array values and return a single array.
- array_multisort() :- Sorts multiple or multi-dimensional arrays.
- array_pop() :- It will delete the last element of an array.
- array_push() :- This function Inserts one or more elements to the end of an array.
- array_rand() :- It will return one or more random keys from the given array.
- array_shift() :- Removes the first element from an array, and returns the value of the removed element.
- array_slice() :- returns selected part of an array.
- array_sum() :- returns the sum of array values.
- in_array() :- check if the given value exists in array.
- sizeof() : – Alternate to count() function.
How to check length of an Array in PHP?
There are two popular functions to check the length of a given array:
- count()
- sizeof()
Examples of checking the length of a PHP array
<?php
$arrayData= array(
‘name’ => ‘John Buttler’,
‘age’ => ’20’,
’email’ => ‘buttler@email.com’
);
echo count($arrayData);
?>
Output will be:- 3
Using PHP loops, you can easily process large arrays of data.
We can iterate over an array using loops, foreach, and do-while, but there is a simpler way. So we will see how we can use the foreach loop to iterate over a large number of array values in a few lines.
<?php
$colors = array(“black”, “red”, “green”);
foreach ($colors as $key=> $value) {
echo “$key $value <br>”;
}
?>
Output will be :
- 0 black
- 1 red
- 2 green
The main point to remember is that the array index always starts at zero. If we count, the length of the array will be three, so don’t get the index value and the length of the array mixed up.
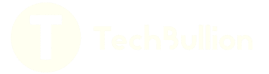