What Is Programming? A Comprehensive Guide to Get Started
Programming, often referred to as coding, is the process of creating instructions that a computer can execute to perform specific tasks. It is a foundational skill in the digital age, enabling the development of software, websites, mobile applications, and even complex systems that drive everything from autonomous vehicles to financial markets. Understanding what programming is and how to get started can open the door to a wide range of opportunities in technology and beyond.
Understanding Programming: The Basics
Programming is the art and science of writing instructions that a computer can understand and execute. These instructions are written in a programming language, which serves as a bridge between human logic and computer operations. At its core, programming involves problem-solving, logic, and creativity, making it both a technical and an intellectual pursuit.
The Essence of Programming
At its simplest, programming is about solving problems. Whether you’re writing a simple script to automate a repetitive task or developing a complex algorithm to analyze data, the goal of programming is to find efficient solutions to real-world problems. This process begins with understanding the problem, breaking it down into smaller, manageable parts, and then devising a sequence of steps (instructions) that can solve the problem when executed by a computer.
Programming languages are the tools used to write these instructions. Just as human languages have rules of grammar and syntax, programming languages have specific syntax and structures that must be followed. These languages range from low-level languages like Assembly, which are closely tied to the machine’s hardware, to high-level languages like Python, which are more abstract and easier for humans to read and write.
The Role of Algorithms
Algorithms are at the heart of programming. An algorithm is a step-by-step procedure for solving a problem or performing a task. For example, an algorithm for sorting a list of numbers might involve comparing each number with the others and arranging them in ascending order. Algorithms can be simple or complex, but they are always designed to be precise and unambiguous so that the computer can follow them without error.
In programming, algorithms are implemented using code. Writing efficient algorithms is a key aspect of programming because it determines how quickly and effectively a program can perform its tasks. Different algorithms can solve the same problem in different ways, and part of becoming a skilled programmer involves learning to choose and implement the most efficient algorithms for a given task.
Different Types of Programming
There are several different types of programming, each serving different purposes:
- Procedural Programming: This is the most basic form of programming, where the program is structured as a sequence of instructions or procedures. It is often used for simple tasks and is characterized by the use of functions and procedures to carry out tasks.
- Object-Oriented Programming (OOP): OOP is a paradigm that organizes programs around objects, which represent real-world entities. Each object can contain data (attributes) and methods (functions) that operate on the data. OOP is widely used in software development because it promotes code reuse and modularity.
- Functional Programming: This paradigm treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. Functional programming is gaining popularity for its ability to handle complex problems with fewer side effects.
- Scripting: Scripting languages like JavaScript, Python, and Ruby are often used for automating tasks, managing systems, and adding functionality to web pages. Scripting is typically more accessible to beginners because it often involves less complex syntax and smaller codebases.
- Declarative Programming: Unlike procedural programming, where you define how to perform a task, declarative programming focuses on what the outcome should be. SQL (Structured Query Language) used in databases is a common example of declarative programming.
How to Get Started with Programming
Getting started with programming might seem daunting, but with the right approach and resources, anyone can learn to code. Here’s a step-by-step guide to help you embark on your programming journey.
1. Choose Your First Programming Language
The first step in learning to program is choosing a programming language. The language you start with should be easy to learn and widely used so that you can apply your skills to various projects. Here are some beginner-friendly languages:
- Python: Python is often recommended for beginners because of its simple syntax and readability. It is a versatile language used in web development, data science, automation, and more.
- JavaScript: If you’re interested in web development, JavaScript is a great choice. It’s the language of the web, used to make websites interactive. It’s also relatively easy to learn and has a large community of developers.
- Ruby: Ruby is known for its elegant syntax that is easy to read and write. It’s often used for web development, particularly with the Ruby on Rails framework.
- Scratch: Scratch is a visual programming language designed for beginners, especially kids. It uses drag-and-drop blocks to create programs, making it easy to understand the basics of programming logic.
Once you’ve chosen a language, you can start learning the basics, such as variables, data types, loops, conditionals, and functions. These are fundamental concepts that apply to all programming languages.
2. Set Up Your Development Environment
To write and run your code, you’ll need a development environment. This typically includes a code editor and a compiler or interpreter that converts your code into machine language.
- Code Editors: A code editor is where you write your code. Some popular code editors for beginners include Visual Studio Code, Atom, and Sublime Text. These editors offer features like syntax highlighting, auto-completion, and debugging tools.
- Integrated Development Environments (IDEs): An IDE combines a code editor with additional tools like debuggers and compilers. IDEs are particularly useful for larger projects. For example, PyCharm is a popular IDE for Python, while Visual Studio is widely used for multiple languages.
- Online Platforms: If you prefer not to install software on your computer, you can use online platforms like Repl.it, Glitch, or CodePen, which provides a web-based environment for writing and running code.
Setting up your development environment is a crucial step because it allows you to test your code and see the results of your programming efforts immediately.
3. Learn the Basics of Programming
Before diving into complex projects, it’s essential to learn the basics of programming. Here are some key concepts you should focus on:
- Variables and Data Types: Variables are used to store data that your program can manipulate. Different types of data, such as numbers, strings, and booleans, require different data types.
- Control Structures: Control structures like loops and conditionals allow your program to make decisions and repeat actions. For example, an if statement can execute a block of code only if a certain condition is true, while a for loop can repeat a task a specified number of times.
- Functions: Functions are reusable blocks of code that perform specific tasks. By defining functions, you can organize your code and avoid repetition.
- Input and Output: Learn how to get input from users and display output. This is often done using functions like print() in Python or console.log() in JavaScript.
- Debugging: Debugging is the process of finding and fixing errors in your code. Learning how to read error messages and use debugging tools is crucial for every programmer.
As you learn these concepts, practice by writing small programs that solve simple problems. For example, you could write a program that calculates the area of a circle or a program that converts temperatures from Celsius to Fahrenheit.
4. Build Projects and Practice Regularly
Once you’ve grasped the basics, the best way to improve your programming skills is by building projects. Projects give you hands-on experience and help you understand how to apply programming concepts in real-world scenarios.
Start with small projects that match your skill level, such as:
- Calculator: Create a simple calculator that can perform basic arithmetic operations like addition, subtraction, multiplication, and division.
- To-Do List: Build a to-do list application where users can add, remove, and mark tasks as complete.
- Weather App: Develop an app that fetches and displays weather data for a given location using an API.
- Game: Create a simple game like Tic-Tac-Toe or Hangman. Games are a fun way to learn about user input, loops, and conditionals.
As you become more confident, you can take on more complex projects, such as a personal blog, an e-commerce website, or a mobile app.
In addition to building projects, practice regularly by solving coding challenges on platforms like LeetCode, HackerRank, and Codewars. These platforms offer problems that range from beginner to advanced levels and allow you to practice algorithms, data structures, and other essential skills.
5. Learn to Use Version Control
As you start working on larger projects, it’s important to learn version control, a system that tracks changes to your code over time. Version control allows you to manage different versions of your code, collaborate with others, and revert to previous states if something goes wrong.
- Git: Git is the most widely used version control system. It allows you to create repositories, track changes, and collaborate with other developers. You can use Git from the command line or through a GUI (Graphical User Interface).
- GitHub: GitHub is a platform that hosts Git repositories online. It’s a great place to store your projects, share your code with others, and contribute to open-source projects.
Learning Git and GitHub is essential for any programmer, especially if you plan to work on collaborative projects or contribute to the open-source community.
6. Join a Community and Seek Help
Programming can be challenging, especially when you encounter bugs or concepts that are difficult to understand. Fortunately, there are many online communities where you can seek help, share your knowledge, and connect with other programmers.
- Stack Overflow: Stack Overflow is a popular Q&A platform where developers ask and answer programming-related questions. If you’re stuck on a problem, chances are someone else has encountered the same issue and posted a solution.
- Reddit: Subreddits like r/learnprogramming and r/programming are great places to discuss programming topics, share resources, and get advice from experienced developers.
- Discord and Slack: Many programming communities have Discord or Slack channels where you can chat with other developers in real-time. These platforms are great for networking and finding collaborators for projects.
- Local Meetups and Coding Bootcamps: If you prefer in-person interaction, consider attending local coding meetups or enrolling in a coding bootcamp. These events provide opportunities to learn from instructors, meet fellow programmers, and work on group projects.
Joining a community not only helps you overcome challenges but also keeps you motivated and inspired as you learn to program.
7. Explore Advanced Topics and Specializations
As you gain experience and confidence in programming, you may want to explore advanced topics and specialize in a particular area. Programming is a vast field with many different branches, including:
- Web Development: Web developers create websites and web applications. Front-end developers focus on the user interface, while back-end developers work on the server-side logic. Full-stack developers handle both.
- Mobile Development: Mobile developers create apps for smartphones and tablets. You can specialize in iOS development using Swift or Objective-C, or in Android development using Java or Kotlin.
- Data Science: Data scientists analyze and interpret complex data to help businesses make informed decisions. This field involves programming languages like Python and R, as well as tools for data analysis, machine learning, and visualization.
- Game Development: Game developers create video games for consoles, PCs, and mobile devices. This field often involves programming languages like C++ and C#, as well as game engines like Unity and Unreal Engine.
- Artificial Intelligence (AI) and Machine Learning (ML): AI and ML developers create systems that can learn and make decisions. This field involves advanced algorithms, neural networks, and programming languages like Python and TensorFlow.
- Cybersecurity: Cybersecurity experts protect systems and data from cyber threats. This field involves knowledge of cryptography, network security, and ethical hacking, as well as programming languages like Python and C.
As you explore these fields, you may decide to pursue a career in one of them or combine skills from multiple areas to create innovative solutions.
The Journey of Becoming a Programmer
Programming is a journey that involves continuous learning, problem-solving, and creativity. Whether you’re building simple scripts or developing complex systems, programming empowers you to bring your ideas to life and make an impact in the digital world.
Getting started with programming requires patience, practice, and perseverance. By choosing the right language, setting up your environment, mastering the basics, building projects, and joining a community, you can develop the skills needed to succeed in the field of programming.
As you progress, you’ll discover new challenges and opportunities, and you’ll have the chance to specialize in areas that interest you most. Remember that programming is not just about writing code, it’s about thinking critically, solving problems, and constantly pushing the boundaries of what’s possible.
Whether you’re looking to start a new career, build your own projects, or simply satisfy your curiosity, programming offers a world of possibilities. The journey may be challenging, but the rewards of creating something meaningful and impactful are well worth the effort.
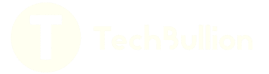