As you begin your programming journey, you will encounter challenges that require you to deal with many instances of the same data types. Typically, arrays will be used for the storing of this data. The larger concern is optimizing the answer for a particular problem to fit within a specific time constraint.
Algorithms, which provide detailed instructions for a specific task, are the basis of computer science. Sorting and searching in Java may seem to be challenging concepts for novices to master, but gaining a solid knowledge of them is very rewarding.
However, some of the most popular, easy, and beneficial Java algorithms facilitate the learning of programming by novices while addressing operational difficulties.
Sort Algorithms
Quick Sort
To put it another way, Quicksort in Java is a sorting algorithm that falls into one of the divides and conquers groups of algorithms. It is an in-place (no need for additional data structures) and non-stable sorting method.
The divide-and-conquer algorithm subdivides a problem into two or more subproblems of the same kind, making them easier to solve. Until an issue is basic enough to be addressed on its own, the breakdown continues (we call this the base case).
This approach has been demonstrated to provide the best possible results when dealing with huge arrays.
Algorithm
- Start
- Select an element.
- Split the problem set and put smaller sections to the left of the pivot.
- Repeat the operations and merge the previously sorted arrays.
- End
Bubble Sort
Bubble sort is the most straightforward and useful algorithm most novices use to begin their sporting careers. This sorting algorithm is inefficient, but they give a crucial understanding of what sorting is and how a sorting algorithm operates in the background. Bubble sort employs several swaps; the algorithm repeatedly traverses the array, exchanging out-of-place elements.
Algorithm
- Start
- Execute two loops, one inner loop, and an outer loop.
- Repeat the instructions until the outer loop is depleted.
- If the current inner loop element is smaller than the next, swap them.
- End
Selection Sort
It is common to use quadratic sorting algorithms since they are simple to learn and implement. These do not provide a unique or optimal method for sorting the array; instead, they should serve as building blocks for those unfamiliar with the idea of sorting. The selection sort employs two loops. The inner loop selects the array’s minimal element and moves it to the index given by the outer loop. Every time the outer loop is executed, one element is relocated to its proper position in the array. It is also a widely used sorting algorithm in Python.
Algorithm
- Start
- Two loops should be executed: an inner one and an outer loop.
- Repeat steps until the minimum elements are found.
- Mark as minimal the element identified by the variable in the outer loop.
- If the inner loop’s current element is less than the minimal element, replace it.
- Swap the minimal element’s value with the element indicated by the outer loop’s variable.
- End
Search Algorithms
Linear Search
The Linear Search method is one of the simplest search algorithms that may be used to discover a particular element within a list of items. The linear search algorithm implements over each item in the list until either a matching item is found or the algorithm reaches the end of the list.
A linear search is used when searching for a crucial element among several components. Because it is slower than binary search and hashing, linear search is less often utilized nowadays.
Linear search algorithm implementation
- Start by iterating over the data in the array.
- Compare the key element to each array member.
- If the key element is discovered, return the array element’s index position.
- Return -1 if the key element could not be located
- End
Binary Search
It is one of the most often utilized search algorithms because of its speedy results. Divide and Conquer are used in this search, and the data collection must be sorted before it can be searched.
As you go through the process, it splits the input set evenly and compares each half to a different one.
It is over when the element is found. If the target element is less or larger than the middle element, we divide and pick the appropriate array. This is why a sorted collection is essential for Binary Search.
Algorithm
- Start
- As a search key, start with the element in the middle of the whole array.
- Return the search key’s index if the key’s value is the same as the item.
- In this case, if the search key’s value is smaller than the middle element in the interval, you may filter the interval down.
- If not, restrict it to the top half.
- To find the value, keep going back and forth between the second and third points.
- End
Conclusion:
Sorting and searching in Java may seem difficult for beginners to learn, but getting a good understanding of either is very beneficial once it is accomplished. However, in this article, we have provided you with a number of the Java algorithms that are now the most popular, straightforward, and advantageous. These algorithms make it easier for beginners to learn how to program while also resolving operational issues.
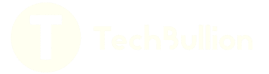