Python is a powerful and versatile programming language that has become increasingly popular in recent years due to its simplicity, ease of use, and wide range of applications. One of the key features of Python is its support for various programming paradigms, including object-oriented, procedural, and functional programming. In this article, we will focus on one of the most fundamental concepts in Python programming, the join method.
Understanding Join in python
The join method Is a built-in function in Python that is used to join strings together. It takes an iterable object (such as a list, tuple, or set) as an argument and returns a string that consists of the elements of the iterable concatenated together with a specified separator. Here’s the basic syntax for the join in python method:
Separator.join(iterable)
In this syntax, separator is the string that will be used to join the elements of the iterable, and iterable is the object that contains the elements to be joined. Let’s look at some examples to see how the join method works in practice.
Example 1: Joining a List of Strings
Suppose we have a list of strings that we want to join together with a comma separator. Here’s how we can do it using the join method:
My_list = [‘apple’, ‘banana’, ‘orange’]
Separator = ‘,’
Result = separator.join(my_list)
Print(result)
Output:
Apple,banana,orange
In this example, we created a list of strings called my_list and a separator string called separator. We then used the join method to concatenate the elements of my_list together with the separator ‘,’. The resulting string was stored in the variable result.
Python joins are a powerful tool that allows you to combine multiple strings into one. They are commonly used in data processing, web development, and other programming applications where it is necessary to combine strings. In this guide, we’ll cover the various Python joins available and how to use them effectively.
Before diving into the different types of joins, it’s important to understand that joining is the process of combining two or more strings into one. Python offers several ways to do this, including concatenation, formatting, and string interpolation. However, these methods can be tedious and error-prone, especially when working with large datasets. Python joins offer a more efficient and streamlined way to combine strings.
There are three main types of Python joins:
Join() method
+ operator
Format() method
- Join() Method
The join() method is a built-in function that takes a list of strings as an argument and joins them into a single string. The syntax is as follows:
Separator.join(iterable)
Here, separator is the string that will be used to separate the elements in the list. For example, if you want to join a list of names with a comma, you would use the following code:
Names = [‘John’, ‘Jane’, ‘James’]
Separator = ‘, ‘
Joined_names = separator.join(names)
Print(joined_names)
Output:
John, Jane, James
In this example, the join() method takes the list of names as an argument and uses the comma and space separator to join them into a single string.
- + Operator
The + operator is another way to join strings in Python. It works by concatenating two or more strings together. For example:
First_name = ‘John’
Last_name = ‘Doe’
Full_name = first_name + ‘ ‘ + last_name
Print(full_name)
Output:
John Doe
In this example, the + operator is used to join the first_name and last_name variables with a space in between.
- Format() Method
The format() method is another way to join strings in Python. It works by replacing placeholders in a string with values from variables. Here’s an example:
Name = ‘John’
Age = 30
Message = ‘My name is {} and I am {} years old.’.format(name, age)
Print(message)
Output:
My name is John and I am 30 years old.
In this example, the format() method is used to replace the {} placeholders in the message string with the name and age variables.
Conclusion
Python joins are a powerful tool for combining strings. Whether you’re working with large datasets or just need to combine a few strings, the join() method, + operator, and format() method can help you do it quickly and efficiently. Understanding how to use these joins effectively can save you time and simplify your code
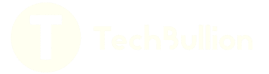