Building a program for a startup can lead developers to face several challenges. Many startups have limited resources and must enter the market as soon as possible or face failure. Thankfully, there are various principles that startups can use that will provide them with a fast and high-quality platform that they can add to as their business grows.
Physical Architecture Versus Software Architecture
Both physical and software architecture concern themselves with computer science, and both are vital to the success of a company. However, there are a few major differences between the two that are crucial to the understanding of how each architecture works.
Physical Architecture
Physical architecture usually deals with the setup of the hardware, like servers and wires. When an individual works with physical architecture, they need to ensure that their plan will satisfy their client’s needs and will last for many years. Although the setup may require some occasional repairs or minor upgrades, it will never need to greatly change. This system will continue to work until it cannot be used anymore.
Software Architecture
Whereas physical architecture concerns itself with physical hardware, software architecture refers to the structure and components of a software system, along with the relationships between them. It can frequently require major changes to satisfy the users’ demands, such as changing from a monolithic layered approach to microservices to support business growth. The needs and habits of clients are constantly changing, and the application must be able to shift to meet these demands. If a platform is not updated to meet these needs, it will become outdated and fail.
The Importance of Domain-Driven Design
Domain-driven design establishes the core focus of a program. This approach centers on programming a domain model with a rich understanding of the domain’s processes and rules. DDD has become a popular approach used by several programmers, and as its popularity continues to grow, so does the need to understand why DDD is so important.
What is a Domain?
To understand DDD, people first must understand what a domain is. In software development, the domain is a set of requirements, terminology, and functionality that defines how a software program solves a problem. In simpler terms, it refers to the purpose of a program. Thus, DDD focuses on developing the core domain.
The Ubiquitous Language
The ubiquitous language is one of the most important parts of DDD. It refers to the common language that is shared between software developers and users. The terms used to describe a component of the program must be specific to that part, as using an ambiguous word can lead to confusion. This allows for more efficient communication and reduces misunderstandings, which is always necessary in a business environment.
The Purpose of DDD
As an application grows, so do the rules, data, and functions it contains. It usually breaks down into various microservices, and without proper organization, the code can turn into an incomprehensible tangle of characters. DDD prevents this by emphasizing the importance of modeling the core domain and using it as a foundation for software design. This allows developers to attain an understanding of the fundamental aspects of the program, which will later help them create software that aligns with the application’s requirements and goals. Overall, DDD helps make a more organized program that solves the startup’s problem in a comprehensible manner.
The YAGNI Principle
YAGNI, which stands for You Ain’t Gonna Need It, is a principle that keeps developers focused on what is immediately necessary. This idea emphasizes adding functionality to a program only when it is certain that the function will be used. Adding a component before it is needed will either result in a completely unnecessary function or will require repairs, which creates a greater cost of time and money that could have easily been avoided.
The SOLID Principles
The SOLID principles establish a baseline of what to do and what not to do in software development. These object-oriented design principles make projects more understandable, flexible, and maintainable. The acronym stands for five rules, including the single-responsibility, open-closed, Liskov substitution, interface segregation, and dependency inversion principles.
Single-Responsibility Principle
“There should never be more than one reason for a class to change.”
A class should not have more than one job. If it has multiple tasks and one requires a change, then it could break the rest of the code and require even more fixes. This can also be applied to microservices, as a microservice responsible for too many functions will also result in errors. Engineers must ensure that a class handles one task and does that task well.
Open-Closed Principle
“Software entities should be open for extension, but closed for modification.”
Classes should be open to new functionality, but the original class cannot be modified. By modifying the original class, bugs may be created. It could also result in a violation of the single-responsibility principle if too many tasks are added to the class. Instead of modifying the original class’s code, engineers should create subclasses to add new functionality to the first class.
Liskov Substitution Principle
“Functions that use pointers or references to base classes must be able to use objects of derived classes without knowing it.”
Any subclass should be able to be used in place of their parent class without affecting the behavior of the parent class. For example, if S is a subtype of T, then objects of type T in a program may be replaced with objects of type S without altering any of the outcomes. Ensure that this principle is followed, as bugs created by breaking this rule can be hard to detect.
Interface Segregation Principle
“Clients should not be forced to depend upon interfaces that they do not use.”
The interfaces that a client uses should be separated and relevant to the client. Instead of having one general interface, there should be multiple smaller interfaces, each with a specific responsibility. This rule is very similar to the single-responsibility principle, but it deals with interfaces instead of classes. Following this rule ensures that the client cannot rely on or use any information that it does not need to know. This is important, as irrelevant information could result in bugs.
Dependency Inversion Principle
“Depend upon abstractions, not concretes.”
High-level modules should not depend on low-level modules. Instead, both should depend on abstractions (interfaces or abstract classes). Furthermore, details should depend on abstractions. This principle may sound complicated, but by successfully applying the open-closed principle and the Liskov substitution principle, the dependency inversion principle is automatically applied as well. Like many of the other principles, this rule reduces the chance of breakage and increases flexibility.
Applying the SOLID Principles to Business
These rules must be followed if a platform is to be successful later on. As a startup grows, the program will become more complex. More code and functions will have to be added to the application, but without proper organization, it will end up breaking and turning into an enormous mess. The SOLID principles are vital to platform flexibility and maintenance, and following them will allow the business to continue successful growth.
The Focus of Unit Testing
Unit testing is a practice that involves isolating and testing the smallest units of code to ensure that each unit performs as desired and meets any requirements. This is very useful, as it can allow developers to catch bugs early and improve code quality. However, engineers should focus on testing the critical parts of the code rather than trying to cover 80% or more of the code. This will save time and allow the program to get released faster, while minor details can be tested and corrected later on. As long as the application can succeed in performing the most critical functions, it can be released.
Getting to the Market Fast
The time it takes for a business to get into the desired market can determine whether it succeeds or fails. If it launches too slowly, other startups will overtake it and leave the business with no clients. Thus, it is important to use simple yet effective programming strategies to quickly establish a platform and get into the market as soon as possible.
A Quick and Efficient Software Architecture
It can be tempting to start with complex approaches like CQRS, microservices, or event sourcing. Engineers may believe that this would simplify platform development later on, but the truth is that this would violate the YAGNI principle, so it would likely cause more problems with the program in the future.
One of the best software architectures to begin with is the layered architecture pattern. In this approach, the application is usually divided into four separate layers: presentation, business, persistence, and presentation. Each layer has a specific role, and each layer can be modified separately from the other tiers. As long as the code remains organized and follows the principles described above, this design will suffice for a small business until it experiences significant growth.
Conclusion
The above principles and strategies will help startups develop a solid platform without spending too many resources. By implementing principles that simplify code and decrease the risk of bugs, startups can focus on growing their business without having to worry about their platform. As the company grows, it can use more complex strategies, but without a solid foundation, it is sure to fail.
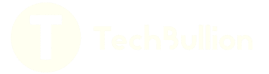