Picture this: you’re knee-deep in a complex Python project, wrestling with intricate logic and a labyrinth of code. Suddenly, an unexpected error rears its ugly head, threatening to derail your progress.
Fear not, my fellow Pythonistas, for Python’s exception handling is here to save the day! In this retrospective analysis, we’ll embark on a captivating journey through the development of Python’s exception handling, uncovering the secrets and best practices that have shaped this indispensable feature.
So, grab your favorite caffeinated beverage, get cozy, and let’s dive into the fascinating world of Python exception handling courtesy of the brilliant minds at CodingViz.com.
The Early Days: Python’s Exception Handling Takes Shape
Imagine when Python was still in its infancy, a mere hatchling in the vast landscape of programming languages.
Even in those early days, the visionary creators of Python recognized the importance of robust exception handling. They set out to craft a system to empower developers to gracefully navigate the treacherous waters of runtime errors.
In Python’s early versions, exception handling was simplified. Developers could use the try and except statements to enclose blocks of code that might raise exceptions.
If an exception occurred within the try block, the program flow would be redirected to the corresponding except block, allowing for targeted error handling. This basic structure laid the foundation for Python’s exception-handling paradigm, setting the stage for future enhancements and refinements.
The Rise of Built-in Exceptions
As Python matured and gained popularity, the need for a comprehensive set of built-in exceptions became apparent. The Python community recognized that having a standardized collection of exceptions would promote consistency and make it easier for developers to handle common error scenarios.
Over time, Python introduced a wide range of built-in exceptions, each tailored to specific error conditions. From the ubiquitous ValueError and TypeError to the more specialized ZeroDivisionError and FileNotFoundError, these exceptions provided a rich vocabulary for expressing and handling various exceptional situations.
Developers could now catch and handle exceptions with greater precision, making their code more robust and maintainable.
The Power of Custom Exceptions
While built-in exceptions cover a wide range of scenarios, developers sometimes need to define their own custom exceptions. Python’s exception-handling system embraced this need, allowing developers to create custom exception classes inherited from the Exception base class.
Developers can encapsulate specific error conditions unique to their application domain by defining custom exceptions. This enables more fine-grained error handling, making the code more expressive and self-documenting.
Custom exceptions can also carry additional information, such as error messages or relevant context, providing valuable insights during debugging and error resolution.
The final Clause: Cleaning Up with Grace
As Python’s exception handling evolved, the final clause emerged as a powerful tool for resource management and cleanup tasks. The finally block, which follows the try and except blocks, is guaranteed to execute regardless of whether an exception occurred or not.
The final clause proved invaluable in scenarios where resources needed to be properly released or cleanup actions had to be performed, such as closing file handles, releasing locks, or terminating network connections.
By encapsulating cleanup logic within the final block, developers could ensure that critical resources were always handled appropriately, even in the face of exceptions.
The with Statement: A New Era of Resource Management
Python’s exception handling took a significant leap forward by introducing the with statement. The statement provided a more concise and intuitive way to manage resources, such as file handles or database connections, while automatically handling the cleanup process.
Using the statement combined with context managers, developers could encapsulate the setup and teardown logic for resources within a single block of code. This not only made the code more readable and maintainable but also eliminated the need for explicit try-finally blocks in many common scenarios.
The with statement became a game-changer in Python’s exception-handling landscape, promoting cleaner and more reliable resource management practices.
Best Practices: Writing Exceptional Python Code
As Python’s exception-handling capabilities evolved, so did the best practices surrounding their usage. The Python community has collectively wisdom has distilled key principles that guide developers in writing robust and maintainable code.
One crucial best practice is to be specific when catching exceptions. Rather than using a bare except clause, which catches all exceptions indiscriminately, developers are encouraged to catch only the exceptions they explicitly intend to handle. This approach prevents unintended suppression of errors and promotes more targeted error handling.
Another important principle is to use exceptions for exceptional situations, not for normal control flow. Exceptions should be reserved for truly exceptional conditions, such as invalid input, resource unavailability, or unexpected system states. Abusing exceptions for regular control flow can lead to convoluted and hard-to-follow code.
The Future of Python Exception Handling
As Python continues to evolve, so does its exception-handling system. With each new version of Python, the language introduces enhancements and refinements to exception handling, making it even more powerful and expressive.
Recent versions of Python have introduced features like exception chaining, which allows exceptions to be linked together to provide a more comprehensive traceback. The traceback module has also been enhanced to offer more detailed information about exceptions, aiding in debugging and error analysis.
Looking ahead, the Python community is actively exploring new ways to improve exception handling. Proposals for features like exception groups and more granular control over exception propagation are being considered, paving the way for even more sophisticated and flexible exception-handling mechanisms.
Takeaway
From its humble beginnings to its current state of sophistication, Python’s exception handling has undergone a remarkable evolution. Through a series of carefully crafted features and best practices, Python has empowered developers to write more robust, maintainable, and expressive code.
As we reflect on the development of Python’s exception handling, we can appreciate the thoughtfulness and dedication of the Python community in shaping this essential aspect of the language.
By embracing the principles of specific exception handling, using exceptions judiciously, and leveraging the power of context managers and the with statement, Python developers can craft elegant and resilient code.
If you’re eager to explore more advanced techniques and best practices in Python exception handling, be sure to check out the wealth of resources available at CodingViz.com. From in-depth tutorials to practical examples, CodingViz.com is your go-to destination for all things Python.
So, fellow Pythonistas, let us raise our keyboards in salute to the remarkable journey of Python’s exception handling. May our code be forever exceptional!
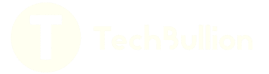