Introduction
You’ve probably heard about OOP (Object Oriented Programming), but you may not know what it is or how it’s different from other programming paradigms. Well, I’m here to change that! This article will help you demystify OOPs Concepts in Java and show you how to apply them in practice.
Inheritance
Inheritance is a mechanism to reuse code. It allows you to create new classes by extending existing classes. You can define the common features of a class and then specialize them in subclasses.
Inheritance is one of the most important concepts in object-oriented programming (OOP). It provides a way to create hierarchies of related classes, where each subclass inherits all the members from its superclass(es). This enables us to model real-world entities using classes that share common properties and behaviors, while still allowing for variation between subclasses based on their specific needs or characteristics.
Encapsulation
Encapsulation is the process of wrapping the data and behavior together in one unit. It’s also known as information hiding, because it hides implementation details from the outside world. If a class encapsulates its data and methods, then we can say that it is “rich” with respect to these two concepts:
- Data: The class contains all information about itself (e.g., its members).
- Behavior: The class defines how it operates on its own state, i.e., what actions do we want this object to perform?
Polymorphism
Polymorphism is a feature of OO languages that allows a variable to be used in more than one way. Polymorphism is used to refer to the ability of objects to behave differently in different contexts.
This means that one method can be inherited from another class, or that an object can have multiple methods with different implementations depending on its type (for example, if you have an Animal class and a Dog class that inherits from Animal).
Abstraction
Abstraction is a way of representing something in a way that is different from the actual thing. For example, when you talk about your friend or colleague, you don’t say “my friend has two arms and two legs.” Instead, you might say “my friend is tall” or “my friend has brown hair.” This means that you are abstracting some of the details about this person so that it’s easier to understand them as an individual.
Information Hiding
In object-oriented programming, information hiding is a concept that allows you to create classes that are easier to maintain and extend. Information hiding refers to the practice of keeping certain details about an object from other parts of the program which need not know about them. This makes it possible for you to change those implementation details without affecting how other parts of your code work with objects from that class.
Information hiding can be done in several different ways:
- Hiding methods: Methods are private by default when you declare them in Java classes, even if they don’t start with an underscore (_) character like this one
Data Hiding
Data hiding is a form of information hiding that is used to hide the implementation details of a class. It’s an important concept in object-oriented programming and can be achieved by declaring fields and methods as private.
Composition vs. Inheritance
Composition is a better way to reuse code.
Composition is a better way to organize code, because it encourages you to think about your application in terms of the objects that make up your domain model rather than as classes and methods. Composition also allows you to define new behaviors for existing classes without having to modify those classes directly, which helps keep them stable over time. Finally, composition allows you to hide implementation details from other parts of your program, making it easier for those parts (and other programmers) to use your code without having direct access or knowledge about how things work under-the-hood.
A quick guide to Object Oriented programming concepts
In the world of programming, object-oriented programming (OOP) is a way of structuring code that helps you create modular and reusable programs. It allows you to define the data, methods and behavior for each individual “object” in your program separately from other objects.
In OOPs Concepts in Java, there are three main concepts:
- Classes – these define what an object looks like and how it operates by defining its properties or attributes as well as its behaviors (methods). They also specify what relationship they have with other classes. For example if we were writing a game where all our characters were animals then we could create two classes: Animal and HumanCharacter which would inherit from another class called CharacterBase;
- Methods – these are functions that perform actions on objects;
- Encapsulation – this means hiding parts of our code so that only certain people can see them or use them
Conclusion
I hope you enjoyed this quick guide to OOP concepts in Java. If you have any questions or comments, please leave them below!
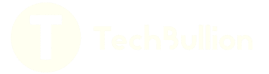