In the domain of modern web development, proficiency in integrating diverse frontend technologies with robust backend platforms is pivotal.
‘Mastering .NET Development: Integrating Angular and React Frontends’ offers a comprehensive guide for developers seeking to enhance their skill set in coupling the powerful .NET ecosystem with two of the most popular frontend frameworks: Angular and React.
This resource delves into the nuanced .NET architecture, providing readers with the foundational knowledge required to set up their development environment effectively.
It further explores the intricacies of melding Angular and React within a .NET context, addressing state management, data flow, and best practices for optimization.
With a focus on real-world applications, this guide is an indispensable tool for developers aiming to build seamless, high-performance web applications.
Understanding .NET Architecture
To effectively integrate Angular and React frontends with .NET, one must first grasp the layered structure of the .NET architecture and its core components. The .NET frameworks, encompassing .NET Core, .NET Framework, and Xamarin, provide a comprehensive runtime environment for developing a variety of applications, from desktop to mobile and cloud-based apps. At the heart of this robust ecosystem is a modular design that encourages the separation of concerns, a principle that enhances maintainability and scalability.
Understanding the intricacies of the .NET frameworks, a developer can leverage the Dependency Injection (DI) design pattern, a fundamental aspect of modern .NET applications. DI is a technique that allows for the decoupling of components, leading to more testable and flexible codebases. By abstracting the dependencies between objects, .NET fosters an environment where diverse frontend technologies can be seamlessly integrated.
Angular and React, each with their distinct ecosystems, can thus be embedded within a .NET application, provided the developer maintains a solid grasp of these underlying principles. It is through this mastery of .NET’s architecture and the strategic implementation of Dependency Injection that a truly cohesive and efficient system can be achieved.
Setting Up Your Environment
Before integrating Angular or React with a .NET application, one must configure the development environment to support these technologies. This preparation is crucial to ensure smooth development and deployment processes. The initial setup involves a series of methodical steps that lay the foundation for a robust Project Structuring and efficient Dependency Management.
When setting up your environment, consider the following:
- Install Node.js and npm: These are essential for running JavaScript tooling and managing front-end dependencies.
- Set up the .NET SDK: Ensure you have the latest version to leverage all features and improvements.
- Choose an IDE: Visual Studio or Visual Studio Code are recommended for their extensive support for .NET, Angular, and React.
- Install Angular CLI/React CLI: These command-line interfaces expedite project scaffolding and build processes.
- Configure your source control: Adopt a system like Git to manage your project’s versioning and collaboration needs effectively.
An experienced developer recognizes that a well-prepared environment reduces friction during the development lifecycle. It’s about aligning tools and workflows to the project’s technical requirements.
With the environment now ready, we can look towards the seamless integration of Angular with .NET, ensuring a cohesive structure that benefits both the development team and the end-users.
Integrating Angular With .Net
Integrating Angular with .NET represents a strategic approach to building robust client-server applications. This process requires a comprehensive understanding of both platforms for effective communication.
The focus here is on establishing a seamless middleware setup that acts as a conduit for data and operations between Angular’s dynamic interfaces and the .NET backend services. This integration ensures smooth communication between the frontend and backend components of the application.
To achieve this, a meticulous configuration of the Angular environment is necessary. This configuration ensures compatibility and optimal performance within the .NET ecosystem.
Angular .NET Communication
We must establish a robust API communication channel to seamlessly integrate Angular with .NET for efficient frontend and backend interaction.Better Developers Angular’s architecture, which includes Angular services and Reactive forms, provides a structured approach for managing data flow and handling form validation.
When Angular services are used in conjunction with .NET’s Web API, a clear protocol for data exchange is required. This integration relies on:
- Crafting well-defined RESTful services in .NET
- Utilizing Angular’s HttpClient to interact with .NET endpoints
- Implementing Reactive forms for responsive user input handling
- Securing API endpoints using authentication and authorization techniques
- Optimizing data serialization and deserialization between Angular and .NET
Seamless Middleware Setup
To ensure a fluid interaction between Angular frontend and .NET backend, one must configure middleware that facilitates the processing of HTTP requests and responses. This integration hinges on adeptly implementing middleware patterns that provide a robust conduit for data exchange.
Middleware in the .NET context acts as a strategic layer where requests can be authenticated, enriched, logged, or even transformed before reaching the core business logic.
A meticulous approach to error handling within this middleware stack is essential. It ensures that any exceptions thrown by the backend do not disrupt the user experience but are instead caught and handled gracefully.
Crafting a seamless middleware setup requires a deep understanding of both the Angular framework and the .NET environment to guarantee that data flows efficiently and securely between the two.
Integrating React With .Net
Transitioning from Angular to React with .NET presents unique challenges and opportunities. Achieving seamless component interoperability within the application architecture is a key challenge. Efficient communication between React components and the .NET backend is paramount. This requires a robust API strategy and thoughtful consideration of data flow and state management.
As we explore this integration, our focus will be on leveraging React’s modular nature. This will enhance the responsiveness and scalability of .NET applications.
React Components Interoperability
Integrating React components into a .NET application requires a clear understanding of both the React library and the .NET framework’s interoperability mechanisms. Component Hydration, a process crucial for attaching event listeners to a server-rendered HTML, is one of the core concepts to grasp.
Furthermore, the interplay between different Library Ecosystems demands a strategic approach to ensure seamless functionality.
- Utilize the ASP.NET Core’s built-in support for server-side rendering of React components.
- Leverage the JavaScriptServices package for bootstrapping React applications within a .NET environment.
- Employ React.NET for more complex scenarios requiring advanced interoperability.
- Ensure synchronization of data flow between the .NET backend and React frontend via RESTful APIs or SignalR.
- Opt for component-based architecture to streamline the development process and enhance maintainability.
NET Backend Communication
Effective communication between a .NET backend and a React frontend is fundamental to the seamless operation of integrated applications. This integration is heavily reliant on the design and implementation of service endpoints. These endpoints must be meticulously crafted to handle requests from the React application and return data in a predictable and efficient manner.
Protocol selection plays a pivotal role in this communication layer, with options such as HTTP/HTTPS being the most common for web applications. Developers must ensure that the data exchange between the .NET backend and React frontend is secure, reliable, and performant.
This requires a deep understanding of both the .NET framework’s capabilities and the React library’s consumption patterns, allowing for a robust integration that leverages the strengths of both technologies.
Managing State and Data Flow
Understanding the nuances of state management is essential when orchestrating data flow between .NET backends and Angular or React frontends. In these ecosystems, state persistence ensures that the user experience remains consistent, while data binding provides a real-time link between the user interface and the underlying data models. This seamless integration is the hallmark of a well-architected application, allowing for dynamic and interactive user experiences.
For developers aiming to master this integration, here are key considerations:
- Single Source of Truth: Maintain a centralized state to ensure consistency and predictability.
- Immutable State Updates: Treat state as immutable to simplify tracking changes and debugging.
- Asynchronous Operations: Handle data fetching, updates, and side effects with robust asynchronous patterns.
- State Synchronization: Implement mechanisms to synchronize state between client and server, avoiding discrepancies.
- Component Lifecycle: Leverage lifecycle hooks effectively to manage state and data flow in response to user interactions and other events.
Best Practices and Optimization
Adopting a set of best practices is crucial for optimizing the performance and maintainability of applications that integrate .NET backends with Angular or React frontends. Performance monitoring should be implemented from the outset, using tools tailored to both .NET and JavaScript ecosystems. This allows developers to identify and address bottlenecks in real-time, ensuring the application runs efficiently under various load conditions. Coupled with this, code refactoring is an ongoing requirement. It is not a one-time task but a continuous effort to enhance code quality and structure.
Analyzing the critical paths within the application, minimizing unnecessary computations, and optimizing data retrieval strategies are key to reducing latency. Developers must rigorously apply principles such as lazy loading, change detection strategies in Angular, and shouldComponentUpdate lifecycle method in React to prevent redundant rendering cycles. These practices directly impact the application’s responsiveness and user experience.
Furthermore, understanding the intricacies of the .NET runtime and JavaScript engines enables developers to write performant code. They must take advantage of the asynchronous programming models available in .NET and the JavaScript event loop to avoid blocking operations that can lead to sluggish interfaces.
Conclusion
In conclusion, adeptly fusing Angular and React with the .NET framework can transform the web development landscape, offering robust, scalable solutions.
Mastery of this integration is akin to having the best of both worlds, providing developers with the flexibility to craft responsive, user-centric applications.
Adherence to best practices and continuous optimization efforts are paramount to ensure the seamless performance and maintenance of such sophisticated software architectures.
The future of .NET development shines bright with such versatile front-end integrations.
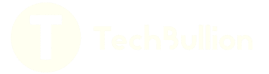