In today’s fast-paced software development landscape, having a reliable system for generating unique identifiers is crucial. One of the most widely recognized forms of these identifiers is the GUID—Globally Unique Identifier. This article will delve deep into the concept of GUIDs, explaining their importance, how they differ from UUIDs, and why developers should consider using an online GUID generator like the online GUID generator or GUID generation tool available today.
What is a GUID?
A GUID (Globally Unique Identifier) is a 128-bit number used to uniquely identify objects, records, or entities across a system, ensuring that no two identifiers are the same—even when generated independently on separate systems. This characteristic is vital for maintaining data integrity in large-scale applications and distributed systems. In essence, a GUID serves as a unique identifier that can be used to reference data without the risk of duplication.
Key Attributes of GUIDs:
- Uniqueness: Each GUID is statistically guaranteed to be unique, making it extremely reliable for differentiating data items.
- Standardized Format: GUIDs typically follow a standard format, such as xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx, where each “x” represents a hexadecimal digit.
- Wide Adoption: They are used in various programming languages and systems including databases, software applications, and network protocols.
A Simple Code Example:
To illustrate, here’s how you might generate a GUID in C#:
using System;
class Program {
static void Main() {
Guid newGuid = Guid.NewGuid();
Console.WriteLine(“Your new GUID is: ” + newGuid.ToString());
This code snippet shows how quickly and easily a new GUID can be generated within a development environment, emphasizing its practical application in ensuring data uniqueness.
GUID vs. UUID: Understanding the Differences
While the terms GUID and UUID (Universally Unique Identifier) are often used interchangeably, there are subtle differences that are worth noting. Both refer to a 128-bit value, but the differences lie primarily in their usage and context.
Similarities:
- 128-bit Structure: Both GUIDs and UUIDs consist of 128 bits.
- Purpose: They are designed to ensure that every identifier is unique across different systems.
- Format: Typically, both follow similar formatting standards (e.g., hexadecimal digits grouped by hyphens).
Differences:
- Terminology:
- GUID: Commonly used in Microsoft environments, especially in Windows-based applications.
- UUID: More broadly used across different platforms and is part of the standard in many open-source projects.
- Generation Algorithms:
- Although both share similar algorithms, some implementations of UUIDs may include timestamp and hardware-based components to enhance uniqueness in distributed systems.
- Use Cases:
- GUIDs are often found in enterprise-level software and database systems, while UUIDs are prevalent in network protocols and open-source systems.
Understanding the nuances between GUID vs. UUID can help developers choose the right tool for their project, ensuring that the identifier they use fits seamlessly within their system architecture.
Common Use Cases for GUIDs
GUIDs play an essential role in various areas of software development. Below, we explore some common scenarios where GUIDs are indispensable.
1. Database Management
In relational databases, GUIDs are used as primary keys for tables. Their uniqueness makes them ideal for ensuring that each record is distinctly identifiable, which is especially useful when records need to be merged from different databases without collision.
2. Distributed Systems
For systems that operate across multiple servers or geographical regions, GUIDs prevent identifier conflicts. Each server can generate a GUID independently, ensuring that identifiers remain unique without the need for central coordination.
3. Software Licensing and Activation
GUIDs are often used to generate unique license keys for software applications. This ensures that each license is unique and can be tracked or validated against a database of issued keys.
4. Session Identification
Web applications utilize GUIDs to create session IDs. This practice enhances security by ensuring that each user session is uniquely identified, making it harder for malicious actors to hijack sessions.
5. Resource Tagging in Cloud Environments
Cloud service providers frequently use GUIDs to tag and manage resources. This allows for efficient tracking, management, and scaling of resources across dynamic environments.
Why Use an Online GUID Generator?
While many programming languages offer built-in libraries for generating GUIDs, using an online GUID generator provides several distinct advantages:
Convenience and Accessibility
An online tool can be accessed from any device with an internet connection, making it ideal for quick testing or generating GUIDs on the fly without the need for a full development environment. Developers can simply visit an online GUID generator and generate a GUID with just a click.
Consistency and Reliability
Online GUID generators are built to conform to the highest standards, ensuring that every GUID generated is truly unique. This reliability is critical when the generated identifiers are used in production systems where collisions can lead to serious issues.
Integration and Testing
For developers involved in rapid prototyping or testing, an online GUID generator can serve as a handy tool to simulate data without having to write additional code. It provides a quick way to generate test data that meets production-level uniqueness criteria.
Educational Value
For those new to the concept, an online tool can offer insights into GUID structure and generation methods. Experimenting with different parameters or formats can provide a deeper understanding of how GUIDs work and why they are structured the way they are.
Example Scenario:
Imagine you are developing a distributed application that requires unique session identifiers for each user. Instead of writing and testing code for GUID generation, you could use an online GUID generator to quickly prototype your user authentication flow. This not only saves time but also ensures that your identifiers adhere to a recognized standard.
Practical Tips for Working with GUIDs
When incorporating GUIDs into your projects, here are a few practical tips to keep in mind:
1. Validate GUIDs
Always validate GUIDs, especially when they are input by users or received from external sources. This can prevent errors caused by malformed identifiers.
2. Use the Right Data Type
Choose the appropriate data type for storing GUIDs in your database. Many systems have a dedicated type for GUIDs which optimizes performance and ensures proper indexing.
3. Avoid Overuse
While GUIDs are excellent for ensuring uniqueness, they may not always be the most efficient choice for primary keys in databases due to their size and randomness. Evaluate your use case carefully to determine if a GUID is necessary or if a simpler numeric ID might suffice.
4. Understand the Trade-offs
The use of GUIDs can sometimes lead to fragmented indexes in databases. Consider strategies such as sequential GUIDs or hybrid approaches if performance is a concern.
5. Security Considerations
Although GUIDs are unique, they are not inherently secure. Avoid using GUIDs for security-sensitive operations like authentication tokens without additional safeguards.
Conclusion
GUIDs are a fundamental element in modern software development, offering a robust solution for ensuring data uniqueness and integrity across diverse systems. Whether you’re managing databases, developing distributed applications, or simply needing a quick way to generate a unique identifier, understanding GUID basics is crucial for any developer.
By comparing GUID vs. UUID, we see that while they serve similar purposes, their contextual applications can vary significantly. Furthermore, using an online GUID generator not only streamlines the development process but also ensures that the GUIDs you implement are reliable and adhere to industry standards.
For developers looking to integrate GUID generation into their projects, leveraging tools like an online GUID generator or a GUID generation tool can save time and improve overall system design. By incorporating these tools, you ensure that your applications are built on a solid foundation of unique, conflict-free identifiers.
Ultimately, the decision to use GUIDs should be informed by a clear understanding of their advantages, limitations, and best practices. Whether you are a seasoned developer or just starting, embracing GUIDs can lead to more robust and scalable systems, empowering you to confidently tackle complex projects.
Embracing the power of GUIDs not only simplifies the development process but also enhances the reliability and scalability of your applications. As you continue to explore the world of unique identifiers, remember that the right tools and practices can make all the difference in creating a secure, efficient, and future-proof system.
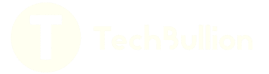