In the modern era, building Chrome extensions is a fun project. Many developers use React for creating the Google Chrome extension. Using this medium, the developer can experiment with new techniques to make the project more interesting. Building the chrome extension with React can be more challenging than you think.
If you need to create a chrome extension using React, you can hire React Developers from www.bosctechlabs.com for your project. With a vast range of experience and in-depth skills, the developer aids you in creating the chrome extension quickly and effectively. Here are step-by-step instructions to build Google Chrome Extension with React:
Overview of Chrome extensions
The Chrome extensions system has various components that interact differently with users and web browsers. For example, the module includes content scripts, UI elements, background scripts, and more.
React – What Is It?
React is an open-source JavaScript library to create UI-based components. It is developed and operated by Facebook. Nowadays, React is mostly used for web development due to its enhanced performance and reusable components.
It allows the process of debugging React web application development quickly. Besides, React provides several extensions for full architectural support of applications like React Native, Flux, and others.
Tips for building a Chrome Extension with React
If you need tips to create the chrome extension using React, you are at the right destination. Here are step-by-step instructions that help beginners to build chrome extensions without difficulty.
1.Build React App
The first step is creating a new React app. Open up the command or terminal prompt and ensure you are in the folder. Then enter the following code to create a new React app.
npx create-React-app React-chrome-ext –template typescript
cd React-chrome-ext
Now you can delete unwanted files and update App.tsx to be React’s fundamental Hello World element. function App() { return ( <div className=”App”> Hello World </div> ); } export default App; Now update index.tsx for building the root element in DOM and add React App. import React from ‘React’; import ReactDOM from ‘React-dom/client’; import App from ‘./App’; const root = document.createElement(“div”) root.className = “container” document.body.appendChild(root) const rootDiv = ReactDOM.createRoot(root); rootDiv.render( <React.StrictMode> <App /> </React.StrictMode> ); Add the following CSS code to App.css: . App { color: white; text-align: center; } .container { width: 15rem; height: 15rem; background-color: green; } |
It will create a new React app in your folder.
2.Set up Webpack
After creating the React App successfully, you should install Webpack. Enter the following commands:
yarn add -D webpack ts-loader webpack-cli
or
npm i -D webpack ts-loader webpack-cli
Then, we can create a webpack.config.js file:
const path = require(“path”);
module.exports = { entry: “./src/index.tsx”, mode: “production”, module: { rules: [ { test: /\.tsx?$/, use: [ { loader: “ts-loader”, options: { compilerOptions: { noEmit: false }, }, }, ], exclude: /node_modules/, }, ], }, resolve: { extensions: [“.tsx”, “.ts”, “.js”], }, output: { filename: “content.js”, path: path.resolve(__dirname, “..”, “extension”), }, }; |
The index.tsx file is the entry point for Webpack. It bundles everything into content.js. The path of our bundled Javascript file is defined as the directory of our Chrome Extension.
By running yarn build, the content.js file will overwrite the existing content script. Now, you can re-load the Chrome extension to apply the modification. The React application should appear on the left side of the website you navigate.
After successfully integrating the React application into an extension, you can use React to build the application you need that works effectively on all web pages.
3.Build a manifest.json file.
You should create a manifest.json file while building the Chrome extension. You can find the manifest.json file generated by Create React Application in the public folder.
Replace everything in this file with the following command:
{
“short_name”: “Your Extension Name”, “name”: “Your Extension Name”, “icons”: { “16”: “favicon.ico”, “48”: “logo192.png”, “128”: “logo512.png” }, “permissions”: [], “manifest_version”: 2, “version”: “0.0.1”, “browser_action”: { “default_popup”: “index.html”, “default_title”: “Your Extension Name” } } |
4.Build a .env File
You should build a .env file in the main folder, which holds the complete environment variables. Add the following code:
INLINE_RUNTIME_CHUNK=false
It will ensure that there are no errors in Google Chrome.
Otherwise, you can add below-given code:
Or add content_security_policy to the manifest.json file:
“content_security_policy”: “script-src ‘self’ ‘unsafe-eval’; object-src ‘self'”
Once you have updated the manifest.json file successfully, you can run the yarn run build script. The script will create a new folder in the current project if successful.
Then, you should add this extension to the Chrome Extensions menu by typing the following code in the newly opened Chrome Tab:
chrome://extensions/
Choose a project’s/build folder:
Reminder: Click on the Update option after saving the code and upgrading it.
Otherwise, you can update a certain chrome extension.
5.Build application
You can run the following command to create a React app.
npm run build
This command generates the output in the build file. If React develops an application, it makes file series for you.
CRA compressed app codes in some JavaScript files for runtime, chunks, main, and others. It will generate a file with all styles and index.html.
CRA develops the App, so you should receive CSP errors. It is well-organized and does not include a lot of JavaScript files. In addition, it automatically inserts some JavaScript code on the HTML pages. Therefore, it is not an error but would not run in Chrome extensions.
6.Load an extension
Now it is time to load the extension into the Chrome browser. Here are some tips for loading an extension:
You should visit chrome://extensions/ on the web browser and allow the developer mode toggle.
Hit on Load unpacked and choose the build file. Now, the extension will load, and you can find it on the list of extensions page.
After that, a new button should appear on the extension’s toolbar. You can see the React demo app by clicking on it.
It would be best if you resized the pop-up as they contain details you need to present to users. Open the index.css file created by React and modify body elements to height and width.
body {
width: 600px; height: 400px; … } |
Return to chrome and see the difference
Conclusion
Building a chrome extension using React is easier than you think. If you set up the chrome extension properly, you can build anything you need effortlessly with React. If you want to build the next project with React technology, and want the customer-centric app for your business then connect with Bosc Tech Labs as who will give your proper guidance and strategies. They also give the estimation of your project which fits into your budget. So let’s connect with us!
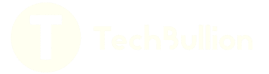