JavaScript is a versatile and powerful programming language that plays a crucial role in web development. As one of the core technologies behind the modern web, JavaScript empowers developers to create dynamic and interactive websites. In this comprehensive guide, we will take a deep dive into the fundamentals of JavaScript, exploring its key features, syntax, and applications.
Understanding JavaScript
JavaScript, often abbreviated as JS, is a high-level, interpreted programming language primarily used for front-end web development. It enables developers to enhance user experience by adding dynamic content, interactivity, and responsiveness to web pages.
- History of JavaScript
JavaScript was created by Brendan Eich in 1995 while he was working at Netscape Communications Corporation. Originally named “Mocha” and later “LiveScript,” it was eventually renamed JavaScript to capitalize on the popularity of Java. Despite the name similarity, JavaScript and Java are distinct languages with different purposes and features.
- Key Characteristics
Interpreted Language: JavaScript is executed by web browsers without the need for compilation, making it an interpreted language. This facilitates rapid development and debugging.
Object-Oriented: JavaScript is object-oriented, supporting features like encapsulation, inheritance, and polymorphism. Objects are fundamental to JavaScript, allowing developers to structure their code in a modular and reusable way.
Event-Driven: JavaScript is event-driven, meaning it responds to user actions and browser events. This makes it ideal for creating interactive and dynamic user interfaces.
Basic Syntax and Structure
- Variables and Data Types
JavaScript uses the var, let, and const keywords to declare variables. It supports various data types, including numbers, strings, booleans, objects, and arrays.
javascript
Copy code
let age = 25; // Number
let name = ‘John’; // String
let isStudent = true; // Boolean
let person = { name: ‘Alice’, age: 30 }; // Object
let numbers = [1, 2, 3, 4, 5]; // Array
- Control Flow
JavaScript supports conditional statements (if, else if, else) and loops (for, while, do-while) for controlling the flow of execution.
javascript
Copy code
let grade = 85;
if (grade >= 90) {
console.log(‘A’);
} else if (grade >= 80) {
console.log(‘B’);
} else {
console.log(‘C’);
}
for (let i = 0; i < 5; i++) {
console.log(i);
}
- Functions
Functions in JavaScript allow developers to encapsulate blocks of code for reuse. They can be defined using the function keyword.
javascript
Copy code
function greet(name) {
console.log(‘Hello, ‘ + name + ‘!’);
}
greet(‘Alice’);
Advanced JavaScript Concepts
- Closures
Closures are a powerful concept in JavaScript, allowing functions to retain access to variables from their outer scope, even after the outer function has finished execution.
javascript
Copy code
function outer() {
let message = ‘Hello’;
function inner() {
console.log(message);
}
return inner;
}
let closureFunction = outer();
closureFunction(); // Outputs ‘Hello’
- Asynchronous JavaScript
JavaScript supports asynchronous programming using callbacks, promises, and async/await. This is crucial for handling tasks like fetching data from a server without blocking the main thread.
javascript
Copy code
// Using Promises
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(‘Data fetched successfully’);
}, 2000);
});
}
fetchData().then((data) => {
console.log(data);
});
- ES6 Features
ECMAScript 6 (ES6) introduced several new features to JavaScript, including arrow functions, template literals, and destructuring assignments.
javascript
Copy code
// Arrow Function
const add = (a, b) => a + b;
// Template Literal
let name = ‘Bob’;
console.log(`Hello, ${name}!`);
// Destructuring Assignment
let person = { firstName: ‘Jane’, lastName: ‘Doe’ };
let { firstName, lastName } = person;
console.log(firstName, lastName);
JavaScript in the Browser
- DOM Manipulation
JavaScript interacts with the Document Object Model (DOM) to dynamically update the content and structure of web pages. This allows developers to create responsive and interactive user interfaces.
javascript
Copy code
// DOM Manipulation
let element = document.getElementById(‘myElement’);
element.innerHTML = ‘New Content’;
- Event Handling
JavaScript enables developers to respond to user actions through event handling. Common events include clicks, keypresses, and form submissions.
javascript
Copy code
// Event Handling
let button = document.getElementById(‘myButton’);
button.addEventListener(‘click’, function() {
console.log(‘Button clicked!’);
});
- AJAX and Fetch API
Asynchronous JavaScript and XML (AJAX) allows for data exchange with a server without reloading the entire page. The Fetch API simplifies making HTTP requests.
javascript
Copy code
// Fetch API
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error(‘Error:’, error));
JavaScript Tools and Libraries
- Package Managers
Tools like npm (Node Package Manager) and yarn simplify the process of managing and installing JavaScript libraries and packages.
bash
Copy code
# Install a package using npm
npm install packageName
- Build Tools
Webpack and Babel are essential tools for bundling, transpiling, and optimizing JavaScript code.
javascript
Copy code
// webpack.config.js
module.exports = {
entry: ‘./src/index.js’,
output: {
filename: ‘bundle.js’,
path: __dirname + ‘/dist’,
},
// …
};
- Popular Libraries and Frameworks
React: A declarative, efficient, and flexible JavaScript library for building user interfaces.
Vue.js: A progressive JavaScript framework for building user interfaces.
Node.js: A server-side JavaScript runtime that allows the execution of JavaScript code outside a web browser.
Conclusion
JavaScript is a fundamental language for web programming, enabling developers to create dynamic and interactive user experiences. This deep dive into JavaScript covered its history, basic syntax, advanced concepts, browser integration, and essential tools and libraries. As web development continues to evolve, a solid understanding of JavaScript is indispensable for any developer aiming to build modern and responsive web applications. Whether you’re a beginner or an experienced developer, mastering JavaScript opens the door to a world of possibilities in web development.
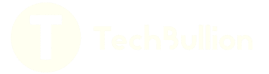