If you use C# often, you will agree with me on this. Most libraries utilise reflection, which can significantly slow down applications and create runtime issues. Some libraries resort to code generators to achieve faster mapping, but this often sacrifices control and adds complexity through the need for rules, configuration files, profiles, and more. Fortunately, C# already provides a quick and easy way to map objects without relying on rules and configuration files, offering full control over every mapping operation. No black magic required. The approach involves using the “implicit” and “explicit” keywords. Let’s take a look at an example.
First, we have a `UserDTO` class:
And a `User` class. For this example, let’s assume we have a `UserDTO` as a data transfer object that needs to be mapped to a `User` class for database storage:
One way to map the `UserDTO` to `User` is by using the constructor in the `User` class. While this approach works, it can lead to duplicated code if you need to perform the mapping multiple times. Luckily, there’s a better solution.
You can leverage implicit and explicit conversion operators in C# to achieve the model-DTO conversions demonstrated above. By using these operators, you eliminate the need for verbose conversion methods, making your code more concise and readable.
Here’s an example of an implicit conversion operator:
A usage sample of how the implicit conversion operator is implemented is shown below:
You can also utilise an explicit conversion operator. It works similar to casting an object as shown below:
Below is a usage example illustrating the above expression.
Please note that you can only define either the implicit or explicit operator, not both. If you define the explicit operator, you can only convert objects explicitly. On the other hand, if you define the implicit operator, you can use it for both implicit and explicit conversions. Although implicit casting is more convenient, explicit casting provides better clarity and enhances code readability.
However, it’s worth mentioning that you can add multiple implicit operators if needed. Here’s an example where we define two implicit operators:
In this case, we have one operator that maps from `UserDTO` to `User` and another that maps to `int`.
Below is a usage example illustrating the explanation provided above:
Finally…
By adopting this approach, you can map objects while maintaining full control. Furthermore, in terms of performance, this method is the fastest way to achieve mapping. Although it requires a bit more work initially, the benefits and rewards become apparent over time. I hope this article was useful to you. Please do not forget to share with friends and in any platform you feel would find the information shared here very useful.
About the Author:
Michael Andifon Etim is a software engineer, mentor, talent coach, and a public speaker with a track record of start-up grooming and advisory. For personal engagement or clarification of any sort of the technical challenge in line with what I shared, please reach me on linkedin via Andifon Etim.
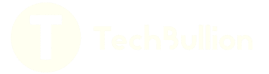